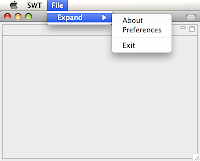
Step 0: Create a HelloWorld RCP application.
Step 1: Add the nested menu actions. Open up the ApplicationActionBarAdvisor class and add the three About, Preferences, and Exit actions as private fields. In makeactions(), define the actions and register them. In the fillMenuBar() method, create two instances of MenuManager, one for the File and one for Expand. Add the three actions to the Expand MenuManager. A Separator is added before the Exit action for the sake of demonstrating this nice menu organizing feature. Next add the Expand MenuManager to the File MenuManager. Finally add the File MenuManager to the menubar.
package com.blogspot.obscuredclarity.nestedmenu; import org.eclipse.jface.action.IMenuManager; import org.eclipse.jface.action.MenuManager; import org.eclipse.jface.action.Separator; import org.eclipse.ui.IWorkbenchWindow; import org.eclipse.ui.actions.ActionFactory; import org.eclipse.ui.actions.ActionFactory.IWorkbenchAction; import org.eclipse.ui.application.ActionBarAdvisor; import org.eclipse.ui.application.IActionBarConfigurer; public class ApplicationActionBarAdvisor extends ActionBarAdvisor { private IWorkbenchAction aboutAction; private IWorkbenchAction preferencesAction; private IWorkbenchAction exitAction; public ApplicationActionBarAdvisor(IActionBarConfigurer configurer) { super(configurer); } protected void makeActions(IWorkbenchWindow window) { //ActionFactory Actions, the ActionFactory defines a set of common actions and can be used in our application. aboutAction = ActionFactory.ABOUT.create(window); register(aboutAction); //register the action so it is deleted when the Workbench window is closed preferencesAction = ActionFactory.PREFERENCES.create(window); register(preferencesAction); exitAction = ActionFactory.QUIT.create(window); register(exitAction); } protected void fillMenuBar(IMenuManager menuBar) { MenuManager fileMenu = new MenuManager("&File", "file"); //create a menuManager to take care of all submenus in "File" MenuManager expandingMenu = new MenuManager("&Expand", "expand"); expandingMenu.add(aboutAction); //Add the "about" action expandingMenu.add(preferencesAction); //Add the "preferences" action expandingMenu.add(new Separator()); //Add a horizontal separator expandingMenu.add(exitAction); //Add the "exit" action fileMenu.add(expandingMenu); //Add the expanding menu to the "File" menu menuBar.add(fileMenu); //Add the "File" menu to the menuBar } }
Step 2: Run the application and test if everything worked. Your application should now have a new menu containing a group of actions nested in a parent menu:

Piece of cake!
<--- Previous - Verify User Intent Before Closing an Eclipse RCP Application
---> Next - Add a Custom Menu Action to an Eclipse RCP Application
Also see: Eclipse RCP Tutorial Table of Contents
4 comments:
Hello Tim,
really good articles, I have followed them and now I understand something about RCP. Really good work, I hope you will continue the tutorials.
I have to do an application with eclipse RCP and I don't know if there is a visual GUI editor in order to complete 20-30 forms. I have installed VE1.4 but it doesn't work with RCP (or I don't know how to use it). There are other comercial plugins like window builder...
Do you use visual GUI editors?
Thanks!
Hi Francisco, Thanks for leaving a comment. I'm glad that my articles are helping someone get started with Eclipse RCP. I have never used any visual GUI editirs with Eclipse. I've only laid out my views by writing the code manually. In the past when I used to create applications with Visual Studio 2005, I at first used the GUI editor, but later coded the GUI by hand because I found I had more control over what was going on.
Learning how to code the layout for SWT widgets can sometimes be frustrating, but it can be done. I found this book to be very helpful. I'll post some tutorials on GUI layout using SWT and JFace soon hopefully. My wife and I just had a baby girl so I don't have as much time as I used to.
By the way, a few friends and I started a new website where we're going to post more Eclipse RCP tutorials and invite others to post their own tutorials as a way to help grow the Eclipse RCP community:
http://eclipsercptutorials.blogspot.com/
Any new tutorials will be added there and not on this blog. You can subscribe to the feed to be notified automatically when new tutorials are posted.
Hi again,
first of all, congratulations for your baby girl!
http://eclipsercptutorials.blogspot.com/ seems to be really interesting.
I'll look for the book that you recommend me.
I'm completly new developing RCP applications. Althought I think the approach of Eclipse its better, I don't know if you have an opinion betwen Eclipse RCP and Netbeans Platform (I have read several comparisons, but I don't know personals opinions about it). In Netbeans Platform the Visual Editor (matisse) seems to work quite well.
What I want is to develope forms, menus, etc in an "agile" way. Do you have experience (or any idea) on developing RCP applications like CRM or ERP? How can you connect the RCP tier with databases (MVC frameworks, hibernate or something like that)?
Too much questions... Answer only if you have enough time ;). (And sorry about my bad English)
Hi Francisco, I know nothing about NetBeans, CRM, or ERP. :(
But I have come across threads in Eclipse RCP forums that discuss using Beans in RCP. The JFace GUI API uses MVC and it's pretty nice. How you connect that to your database I don't know (yet). But I'm sure it's pretty common practice.
You should check out this RCP forum and ask your questions there, or find threads that already answer your questions: http://www.eclipse.org/newsportal/thread.php?group=eclipse.platform.rcp
Your English is awesome! I'm learning German.
Stop by again sometime...
Post a Comment